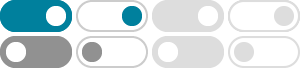
How to Iterate Over Characters of a String in JavaScript
Nov 12, 2024 · There are several methods to iterate over characters of a string in JavaScript. 1. Using for Loop. The classic for loop is one of the most common ways to iterate over a string. …
for/in loop with string in JavaScript - Stack Overflow
Oct 24, 2013 · In javascript for..in works this way: console.log(key, objectOrArray[key]); Hence it always outputs the key, which is the character index (0 based) when looping through a string. …
How to Iterate Through Strings in JavaScript - Medium
Apr 22, 2020 · In order to demonstrate the processing of the single characters of our string, we’ll use the following function: for loop The classic approach — a simple for loop:
JavaScript For Loop - W3Schools
JavaScript supports different kinds of loops: The for statement creates a loop with 3 optional expressions: Expression 1 is executed (one time) before the execution of the code block. …
How to iterate through a string in JavaScript - Suraj Sharma
In this tutorial, you will learn two ways to iterate through a string in JavaScript. Using for loop statement. String.CharAt() function is use to get the string's character at index i. Example …
A Comprehensive Guide to String Iteration Methods in JavaScript
Nov 14, 2023 · 3. Use in Loops. You can consume the iterator in for-of loops: for (let char of str) { console.log(char); } // Prints characters. Or with a while loop: while(!iterator.done) { …
Traverse a string in JavaScript - Techie Delight
One way is to use a for loop with the length property and the charAt() function of the string object. This function will loop through the string from the first character to the last one, using an index …
How to Parse Strings in JavaScript
Jun 23, 2023 · In this post, we will be looking at the following 3 ways to parse strings in JavaScript: By using String.split() method; By using Regular Expressions with RegExp.exec() …
How to split a comma separated string and process in a loop using ...
Jul 14, 2010 · Example using async/await in a forEach loop: const files = "name1,name2,name3" if (files.length) { await forEachAsync(files.toString().split(','), async (item) => { console.log(item) });
Iterating Over Each Character in a JavaScript String Efficiently
Dec 12, 2024 · In this article, we'll explore some efficient approaches to iterating over characters in a JavaScript string. The for loop is one of the most traditional and commonly used methods …