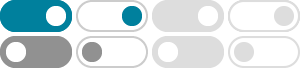
comparison operators - Difference between == and === in …
Feb 7, 2009 · === and !== are strict comparison operators: JavaScript has both strict and type-converting equality comparison. For strict equality the objects being compared must have the same type and: Two strings are strictly equal when they have the same sequence of characters, same length, and same characters in corresponding positions.
What does the !! (double exclamation mark) operator do in …
The == and != operators do type coercion before comparing. This is bad because it causes ' \t\r\n' == 0 to be true. This can mask type errors. JSLint cannot reliably determine if == is being used correctly, so it is best to not use == and != at all and to always use the more reliable === and !== operators instead.
How do you use the ? : (conditional) operator in JavaScript?
Jun 7, 2011 · If you see any more funny symbols in JavaScript, you should try looking up JavaScript's operators first: Mozilla Developer Center's list of operators. The one exception you're likely to encounter is the $ symbol. To answer your question, conditional operators replace simple if statements. An example is best:
Which equals operator (== vs ===) should be used in JavaScript ...
Dec 11, 2008 · JavaScript has two sets of equality operators: === and !==, and their evil twins == and !=. The good ones work the way you would expect. If the two operands are of the same type and have the same value, then === produces true and !== produces false. The evil twins do the right thing when the operands are of the same type, but if they are of ...
Where would I use a bitwise operator in JavaScript?
Mar 17, 2009 · All this 20 lines or so of examples could have been expressed in a single sentence: The bitwise operators work with 32-bit, two's complement big-endian (32-bit, for short) representations of numbers, so any of their operands is converted to this format according to the following rules: a number is converted from the IEEE-754 64-bit format to 32-bit and anything else is first converted to a ...
Is there a "null coalescing" operator in JavaScript?
Jan 25, 2009 · beware of the JavaScript specific definition of null. there are two definitions for "no value" in javascript. 1. Null: when a variable is null, it means it contains no data in it, but the variable is already defined in the code. like this:
javascript - What is the difference between the `=` and `==` …
I would check out CodeCademy for a quick intro to JavaScript. If you prefer to read more, MDN is a great intro as well. For those concerned about the source of the term "identity operator" jbabey pointed out that JavaScript: The Definitive Guide seems to mention it.
JavaScript comparison operators: Identity vs. Equality
Aug 9, 2016 · I've been trying to understand the difference between JavaScript's comparison operators: identity and equality. From what I've read, if you check the equality of two objects using ==, JavaScript will try to figure out if they are the same type and, if not, try to get them to that same type. However, === doesn't behave in the same manner.
What's the difference between & and && in JavaScript?
@Bludream: The explanation in this answer is wrong. You do not use bitwise operators for logical (boolean) comparisons. You use logical (boolean) operators. They do different (although similar) things, expect different inputs, produce different outputs, and have different operator precedence. –
operators - What do ">>" and "<<" mean in Javascript? - Stack …
Aug 9, 2011 · These are the shift right and shift left operators. Essentially, these operators are used to manipulate values at BIT-level. They are typically used along with the the & (bitwise AND) and | (bitwise OR) operators and in association with masks values such as the 0x7F and similar immediate values found the question's snippet.