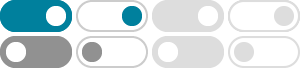
How do I add five numbers from user input in Python?
s = 0 for i in range(5): s += int(raw_input('Enter a number: ')) Second, you can simplify it using the built-in sum function: s = sum(int(raw_input('Enter a number: ')) for i in range(5))
Python's sum(): The Pythonic Way to Sum Values
In this step-by-step tutorial, you'll learn how to use Python's sum() function to add numeric values together. You also learn how to concatenate sequences, such as lists and tuples, using sum().
python - Function to sum multiple numbers - Stack Overflow
Apr 26, 2018 · There are several ways how to add a different quantity of numbers. First of all, you can just use a list and built-in function sum: sum([1, 2, 3]) If you wouldn't like to use a list, try to …
How do I add together integers in a list (sum a list of numbers) in python?
Dec 17, 2012 · x = [2, 4, 7, 12, 3] sum_of_all_numbers= sum(x) or you can try this: x = [2, 4, 7, 12, 3] sum_of_all_numbers= reduce(lambda q,p: p+q, x) Reduce is a way to perform a …
How to Add Two Numbers in Python - W3Schools
Learn how to add two numbers in Python. Use the + operator to add two numbers: In this example, the user must input two numbers. Then we print the sum by calculating (adding) the …
Write a Python Program to Add N Numbers Accepted from the …
May 14, 2024 · In Python, you can achieve this by writing a program to add n numbers accepted by the user using the with and without functions. I will be explaining both the approach here. …
Various ways to sum numbers in Python - Flexiple
Mar 14, 2022 · The sum of numbers can be obtained in python using the in-build function sum (), by using for loop, or using recursive function. The easy way to sum numbers is by using the …
Efficient Numerical Calculations in Python
This article provides a comprehensive guide on adding 5 numbers in Python, exploring theoretical foundations, practical applications, step-by-step implementation, and real-world use cases.
How to Add Numbers in Python: A Comprehensive Guide
Dec 27, 2023 · In this comprehensive, 2500+ word Python programming tutorial, we‘ll explore the ins and outs of adding numbers in Python. You‘ll learn: Using the + operator for basic addition; …
Write the program in python to print the sum of 5 numbers
Mar 18, 2021 · Use a loop to take 5 numbers as input from the user: a. Inside the loop, use the input() function to take a number as input and convert it to an integer using the int() function. b. …