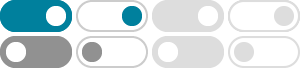
python - Delete a column from a Pandas DataFrame - Stack …
Nov 16, 2012 · As Wes points out in his answer, del df['column'] maps to the Python magic method df.__delitem__('column') which is implemented in Pandas to drop the column. …
python - How to delete a column from a data frame with pandas?
Jan 20, 2015 · The best way to delete a column in pandas is to use drop: df = df.drop('column_name', axis=1) where 1 is the axis number (0 for rows and 1 for columns.) To …
python - how do I remove rows with duplicate values of columns …
Jun 16, 2018 · import pandas as pd data = pd.read_excel('your_excel_path_goes_here.xlsx') #print(data) data.drop_duplicates(subset=["Column1"], keep="first") keep=first to instruct …
What is the best way to remove columns in pandas [duplicate]
The recommended way to delete a column or row in pandas dataframes is using drop. To delete a column, df.drop('column_name', axis=1, inplace=True) To delete a row, df.drop('row_index', …
python - How to drop rows from pandas data frame that contains …
If your string constraint is not just one string you can drop those corresponding rows with: df = df[~df['your column'].isin(['list of strings'])] The above will drop all rows containing elements of …
python - Removing header column from pandas dataframe - Stack …
Apr 18, 2016 · Removing header column from pandas DataFrame. It can be done without writing out to csv and then reading again. Solution: Replace the column names by first row of the …
python - Remove Unnamed columns in pandas dataframe - Stack …
May 15, 2017 · NOTE: very often there is only one unnamed column Unnamed: 0, which is the first column in the CSV file. This is the result of the following steps: This is the result of the …
python pandas remove duplicate columns - Stack Overflow
Feb 20, 2013 · If it is False then the column name is unique up to that point, if it is True then the column name is duplicated earlier. For example, using the given example, the returned value …
Python Pandas: drop a column from a multi-level column index?
Aug 5, 2014 · With a multi-index we have to specify the column using a tuple in order to drop a specific column, or specify the level to drop all columns with that key on that index level. …
python - Drop columns if rows contain a specific value in Pandas ...
Jun 25, 2019 · if df.loc[df['A'] == 'salty']: df.drop(df.columns[0], axis=1, inplace=True) But I am quite lost at finding documentation onto how to delete columns based on a row value of that …