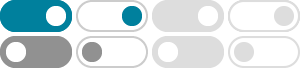
javascript - Linked list with recursion - Stack Overflow
Dec 12, 2020 · There are several issues in your implementation: let newNode = new Node(this.add(data)); This will just keep calling itself without end. The code that you have …
Search an element in a Linked List (Iterative and Recursive)
Feb 18, 2025 · Search an element in a Linked List (Recursive Approach) - O (N) Time and O (N) Space The idea is to recursively traverse all the nodes starting from the head of linked list.
Search for Elements in a Linked List using Recursive Approach
In this article, we will use the recursive approach to search for an element in the linked list. The search method takes the current node curr_node of the linked list, the value to search, and an …
Search Element in a Linked List using JavaScript
To search for an element in a linked list, we need to go through each node one by one and check if its value matches the target element. If we find the element, we return its position in the list. …
JavaScript: Recursive function used to find a Linked list node …
Feb 5, 2016 · Your function should be like this: function getItem(city, item) { if(item === null) return null; if (item.element === city) return item; return getItem(city, item.next) } This is the general …
Recursive Approach to find nth node from the end in the linked …
Jul 31, 2022 · Find the nth node from the end in the given linked list using a recursive approach. Examples: Input : list: 4->2->1->5->3 n = 2 Output : 5 Algorithm: findNthFromLast(head, n, …
Basic Linked List Implementation in JavaScript
Sep 21, 2024 · hi - The Odin Project uses linked lists for dealing with collisions in Hash Map. Each bucket will have a linked list, and add a node every time there's a collision.
Traversing Linked Lists In Javascript | by VTECH - Medium
May 3, 2022 · Linked list traversal allows us to get from the first node (head) to the last node and eventually the Null value. We cannot traverse the linked list from any random node, it must …
LinkedList recursion problem using Javascript - Stack Overflow
Jan 16, 2019 · 1) Store a reference to current (initially head), next (initially null), previous (initially null) node. 2) Set tail to head, if your using a tail pointer. 3) Traverse this list setting next to …
Javascript Program For Writing A Function To Get Nth Node In A Linked …
Jul 31, 2023 · Write a GetNth () function that takes a linked list and an integer index and returns the data value stored in the node at that index position. Example: