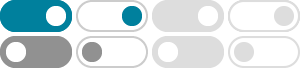
A Python Guide to the Fibonacci Sequence
In this step-by-step tutorial, you'll explore the Fibonacci sequence in Python, which serves as an invaluable springboard into the world of recursion, and learn how to optimize recursive …
Python Program to Display Fibonacci Sequence Using Recursion
In this program, you'll learn to display Fibonacci sequence using a recursive function.
Print the Fibonacci sequence – Python | GeeksforGeeks
Mar 22, 2025 · Using Recursion . This approach uses recursion to calculate the Fibonacci sequence. The base cases handle inputs of 0 and 1 (returning 0 and 1 respectively). For …
python - Efficient calculation of Fibonacci series - Stack Overflow
Aug 11, 2013 · There are a few options to make this faster: 1. Create a list "from the bottom up" The easiest way is to just create a list of fibonacci numbers up to the number you want. If you …
Fibonacci Series in Python using Recursion - Python Examples
Learn to generate the Fibonacci series in Python using recursion. Explore two methods, comparing brute force and optimized recursive approaches.
Multiple Recursive Calls: Fibonacci Sequence, Part 1
When we ask for fib(n) we are asking for the place that nth number occupies in the Fibonacci sequence, similar to if we asked for the 10th prime number, or the 6th triangular number. If we …
Fibonacci Series in Python | Code, Algorithm & More - Analytics …
Oct 24, 2024 · Fibonacci numbers recursively in Python using recursive features. Here’s a Python code to calculate the nth Fibonacci number by using recursion: Def fibonacci(n): if n <= 0: …
Fibonacci sequence with Python recursion and memoization
Jun 16, 2019 · The Fibonacci sequence is a sequence of numbers such that any number, except for the first and second, is the sum of the previous two. For example: [0, 1, 1, 2, 3, 5, 8, 13, …
Implementing the Fibonacci Sequence in Python - PerfCode
Sep 7, 2024 · Learn how to implement the Fibonacci sequence in Python using recursion, iteration, dynamic programming, and the closed-form expression, suitable for both beginners …
Fibonacci in python, recursively into a list - Stack Overflow
Jun 14, 2016 · In Python 3 you can do an efficient recursive implementation using lru_cache, which caches recently computed results of a function: from functools import lru_cache …