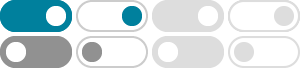
Simplest/cleanest way to implement a singleton in JavaScript
class Singleton { constructor() { this.message = 'I am an instance'; } } module.exports = new Singleton(); Please note that we export not the class Singleton but instance Singleton(). …
How can I change an element's class with JavaScript?
2. Remove class. Method 1 - The best way to remove a class from an element is the classList.remove() method.. Case 1: Remove single class
javascript: what's the difference between a function and a class
Aug 15, 2012 · JavaScript treats functions as first-class objects, so being an object, you can assign properties to a function. Hoisting is the JavaScript interpreter’s action of moving all …
How to document ECMA6 classes with JSDoc? - Stack Overflow
Jan 18, 2017 · }, JSDoc ignores them. furthermore, the only way it recognizes classes is if i declare them as objects: const MyClass = class { ... I have been struggling so badly to …
javascript - Declaring static constants in ES6 classes? - Stack …
Sep 18, 2015 · class Example { static const constant1 = 33; } But unfortunately this class property syntax is only in an ES7 proposal, and even then it won't allow for adding const to the …
Static variables in JavaScript - Stack Overflow
Oct 8, 2009 · The simplest solution I found: don't define a static variable in the class at all. When you want to use a static variable, just define it there and then, e.g. someFunc = => { …
JavaScript class Instance vs Static - Stack Overflow
Aug 18, 2020 · In class instance example, 2 objects are created, the original class and a clone also using new. (If there is a situations where multiple processes with different data need to …
Javascript export/import class - Stack Overflow
If you want to call a method as a class method (without creating an object instance) you can try static methods. You can change the file2.js as, export default class Example { static test() { …
javascript - ES6 Classes for Data Models - Stack Overflow
Jan 31, 2016 · I'm trying to use ES6 Classes to construct data models (from a MySQL database) in an API that I'm building. I prefer not using an ORM/ODM library, as this will be a very basic, …
javascript - ES6 Class Multiple inheritance - Stack Overflow
Apr 26, 2015 · class Example { constructor (props) { Object.assign(this, new Class1(props)) Object.assign(this, new Class2(props)) } } Another way you should consider. In this example …