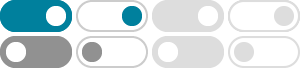
Recursive Programs to find Minimum and Maximum elements of array
Sep 19, 2023 · Given an array of integers arr, the task is to find the minimum and maximum element of that array using recursion. Examples : Output: min = -5, max = 8. Input: arr = {1, 4, …
C recursive program to find the maximum element from array
Jul 9, 2017 · You can change your code like this to have a recursive, stateless function that can determine the maximum value of the array. int find_maximum(int a[], int n) { return …
c - Find max in array with recursion - Stack Overflow
Mar 20, 2015 · if(a[n-1]>m_max) m_max=a[n-1]; return m_max; // add } This linear approach gives recursion a bad name. If there are n numbers, the maximum recursive depth is n .
Finding Max value in an array using recursion - Stack Overflow
Oct 25, 2013 · return Math.max(a[index], findMax(a, index-1)) } else { return a[0]; This much better shows what is going on, and uses the default "recursion" layout, e.g. with a common base …
Find Minimum and Maximum Elements of Array Using Recursion in C++
Nov 3, 2021 · Learn how to find minimum and maximum elements of an array using recursive functions in C++. This guide provides step-by-step explanations and code examples.
C++ Program to Find the Minimum and Maximum Element of an Array
Jan 17, 2023 · We can use min_element () and max_element () to find minimum and maximum of array. Output: Time Complexity: O (n) Auxiliary Space: O (1), as no extra space is used.
Program to Find Maximum Number From Array Using Recursion
Dec 30, 2024 · Define a function find_max(array, n) where: array is the array of numbers. n is the size of the array or the current index being processed. Base Case: If n == 1, return array[0]. …
C++ Recursion: Maximum and minimum elements in an array
Apr 14, 2025 · Write a C++ program to recursively find the maximum and minimum elements in an array by splitting the array into subarrays. Write a C++ program that implements a recursive …
How to Find Maximum Value in Array in C++ | Delft Stack
Feb 12, 2024 · Find Maximum Value in an Array in C++ Using the Recursive Approach. A recursive approach to finding the maximum value in an array provides an alternative method, …
Find maximum and minimum elements in array using recursion
Nov 29, 2020 · Here is the source code of the C++ Program to Find maximum and minimum elements in an array using recursion.