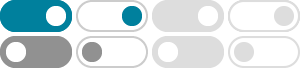
Assign Function to a Variable in Python - GeeksforGeeks
Feb 26, 2025 · To assign a function to a variable, use the function name without parentheses (). If parentheses are used, the function executes immediately and assigns its return value to the variable instead of the function itself. Syntax: Example 1: …
python - Assigning a function to a variable - Stack Overflow
Jan 3, 2023 · When you assign a function to a variable you don't use the but simply the name of the function. In your case given def x(): ..., and variable silly_var you would do something like this: silly_var = x and then you can call the function either with . x() or. silly_var()
Passing function as an argument in Python - GeeksforGeeks
Mar 1, 2025 · By passing a function as an argument, we can modify a function’s behavior dynamically without altering its implementation. For Example: Explanation: process () applies a given function to text and uppercase () converts text to uppercase. Passing uppercase to process with “hello” results in “HELLO”.
Python Functions - W3Schools
To call a function, use the function name followed by parenthesis: Information can be passed into functions as arguments. Arguments are specified after the function name, inside the parentheses. You can add as many arguments as you want, just separate them with a comma. The following example has a function with one argument (fname).
Set function signature in Python - Stack Overflow
Sep 11, 2009 · # define a function def my_func(name, age) : print "I am %s and I am %s" % (name, age) # label the function with a backup name save_func = my_func # rewrite the function with a different signature def my_func(age, name) : # use the backup name to use the old function and keep the old behavior save_func(name, age) # you can use the new signature ...
Python assign a function to another function - Stack Overflow
May 9, 2013 · Can use "getattr" to get the function using the string name of the function (A function is an object). Then you can change the name and call / call something else (original named function) in the new named call.
Python Functions are First-Class Objects: How to Assign, Pass, …
Assign functions to variables in Python by using the function name without parentheses. This creates a reference to the function that can be called later using the variable name with parentheses. hello = greet print ( hello ( 'Alice' )) # Hello, Alice!
Assigning a variable name to a function - scipython.com
Assigning a variable name to a function In Python, functions are "first class'' objects : they can have variable names assigned to them, they can be passed as arguments to other functions, and can even be returned from other functions.
How to Assign a Function to a Variable in Python? - Finxter
Dec 14, 2020 · Method 1: Assign Function Object to New Variable Name. A simple way to accomplish the task is to create a new variable name g and assign the function object f to the new variable with the statement f = g. Here’s the code snippet that solves the problem: # Method 1 def f(): print('Finxter') # Assign function f to g g = f # Run g g()
Functions in Python – Assigning Functions to Variables
Apr 30, 2022 · If you assign a function to a variable, you can use the variable as the function: def double(number): return number * 2 print(double(5)) a = "abc" print(a) a = double print(a(17)) b = a print(b(12))