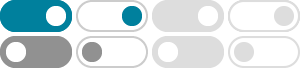
How to fetch input from the csv file in python - Stack Overflow
Aug 9, 2018 · Use the pandas module to read the file into a DataFrame. There is a lot of parameters for reading csv files with pandas.read_csv. The dataframe.to_string() function is extremely useful. Solution: # import module with alias 'pd' import pandas as pd
Working with csv files in Python - GeeksforGeeks
Aug 7, 2024 · Reading from a CSV file is done using the reader object. The CSV file is opened as a text file with Python’s built-in open () function, which returns a file object. In this example, we first open the CSV file in READ mode, file object is converted to csv.reader object and further operation takes place. Code and detailed explanation is given below.
Reading and Writing CSV Files in Python – Real Python
In this article, you’ll learn how to read, process, and parse CSV from text files using Python. You’ll see how CSV files work, learn the all-important csv library built into Python, and see how CSV parsing works using the pandas library. So let’s get started!
csv — CSV File Reading and Writing — Python 3.13.3 …
1 day ago · csv. reader (csvfile, dialect = 'excel', ** fmtparams) ¶ Return a reader object that will process lines from the given csvfile. A csvfile must be an iterable of strings, each in the reader’s defined csv format. A csvfile is most commonly a file-like object or list. If csvfile is a file object, it should be opened with newline=''.
Python CSV: Read & Write CSV Files (With Examples) - Datamentor
Python provides a built-in module named csv that makes working with csv files a lot easier, and to use it, we must first import it. After importing, we can perform file operations like Reading and Writing on the csv file. 1. Read CSV File. The csv module provides a reader() function to perform the read operation.
Python CSV File Handling: Basics, Examples, and Troubleshooting
Python provides multiple ways to read CSV files, but the built-in csv is most common and simple approach. Here is a sample Python script to read a CSV file using the in-built csv.reader module: Now let us see a sample script that uses the csv.DictReader module: You can write or create or write data to a CSV file using csv.writer () module.
Python code to read CSV file based on user input
May 28, 2019 · fileinput = str(input("Which file do you want?")) fileinput += ".csv" You'll want to import it as a pandas dataframe so you can easily call rows and columns. Then you can use iloc to return a row or column in the dataframe. rowcol = input("Column or row?") column = int(input("Which column?")) result = data.iloc([column])
Python CSV: Read And Write CSV Files • Python Land Tutorial
Dec 6, 2022 · Learn how to read and write CSV files with Python using the built-in CSV module or by using Numpy or Pandas. With lot's of example code.
Reading Rows from a CSV File in Python - GeeksforGeeks
Dec 20, 2021 · To read data row-wise from a CSV file in Python, we can use reader and DictReader which are present in the CSV module allows us to fetch data row-wise. Using reader we can iterate between rows of a CSV file as a list of values.
Reading CSV files in Python - Programiz
We have already covered the basics of how to use the csv module to read and write into CSV files. If you don't have any idea on using the csv module, check out our tutorial on Python CSV: Read and Write CSV files. Let's look at a basic example of using csv.reader() to refresh your existing knowledge.