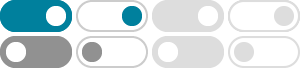
when to use functions in python and when not to ... - Stack Overflow
Aug 12, 2018 · Functions are a way of compartmentalizing code such that it makes it both easier to read and easier to manage. In this case, there are a few concepts you must understand before implementing functions to solve your problem. Functions follow the format:
python - Why do some functions have underscores - Stack Overflow
May 24, 2024 · In Python, the use of an underscore in a function name indicates that the function is intended for internal use and should not be called directly by users. It is a convention used to indicate that the function is "private" and not part of the public API of the module.
python - How can I use a global variable in a function? - Stack …
Jul 11, 2016 · In Python, the module is the natural place for global data: Each module has its own private symbol table, which is used as the global symbol table by all functions defined in the module. Thus, the author of a module can use global variables in the module without worrying about accidental clashes with a user’s global variables.
python - How do I call a function from another .py file ... - Stack ...
#Import defined functions from myfun import * #Call functions Print_Text() c=Add(1,2) Solution2: if this above solution did not work for Colab. Create a foldermyfun; Inside this folder create a file __init__.py; Write all your functions in __init__.py; Import …
In Python, when should I use a function instead of a method?
The Zen of Python states that there should only be one way to do things- yet frequently I run into the problem of deciding when to use a function versus when to use a method. Let's take a trivial example- a ChessBoard object.
python - How to use a variable defined inside one function from …
python_file1.py x = 20 def test(): global x return x test() python_file2.py from python_file1 import * print(x) Solution 2: This solution can be useful when the variable in function 1 is not predefined.
python - Using a dictionary to select function to execute - Stack …
@Ben Believe me, I am very aware of that, I have 8 years providing software solutions, this is the first time I use Python and I wish I could use many security features from other languages, however I can not control it, I need to make the module somewhat "Read-Only", the costumer asks us to give them "proofs" that the scripting feature is as ...
python - What does ** (double star/asterisk) and * (star/asterisk) …
Aug 31, 2008 · BONUS: From python 3.8 onward, one can use / in function definition to enforce positional only parameters. In the following example, parameters a and b are positional-only , while c or d can be positional or keyword, and e or f are required to be keywords:
calling a function from class in python - different way
IMHO: object oriented programming in Python sucks quite a lot. The method dispatching is not very straightforward, you need to know about bound/unbound instance/class (and static!) methods; you can have multiple inheritance and need to deal with legacy and new style classes (yours was old style) and know how the MRO works, properties...
python - How do I forward-declare a function to avoid …
Python's functions are anonymous just like values are anonymous, yet they can be bound to a name. In the above code, foo() does not call a function with the name foo, it calls a function that happens to be bound to the name foo at the point the call is made. It is possible to redefine foo somewhere else, and bar would then call the new function.