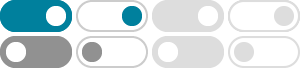
I want to make a program that calculate a circle area in OOP style …
from circle import Circle circle1 = circle(30) print(circle1.cirArea()) circle.py class Circle: def __init__(self, radius): self.radius = radius def cirArea(self): return self.radius * self.radius * 3.14
Python OOP: Circle class with area and perimeter calculation
Apr 21, 2025 · Write a Python class for Circle that includes a class-level constant for π, and implement comparison operators to compare circles based on area. Write a Python class that …
Python Program to Find the Area and Perimeter of the Circle
Write a Python program to calculate the area of a circle and perimeter using math modules and classes with detailed explanations and examples.
Create a Class to Compute Area and Perimeter of a Circle in Python
Mar 11, 2021 · Learn how to create a class in Python that computes the area and perimeter of a circle. Step-by-step guide with examples.
Python program to find the area and circumference of Circle
Write a python program that has a class named Circle. Use a class variable to define the value of a constant PI. Use this class variable to calculate the area and circumference of a circle with …
Python Program to Create a Class and Compute the Area and the …
Apr 26, 2024 · Below is the full approach for writing a Python program that creates a class with two methods that calculate the area and perimeter of the circle. Approach: Give the radius of …
26. Problem to calculate area of circle using class in Python
In this Python oops program, we will create a example to calculate area of circle using class in Python. This code demonstrates the basic functionality of the Circle class, allowing you to …
Understanding Python Class Attributes By Practical Examples
Outside the Circle class, you can access the pi class attribute via an instance of the Circle class or directly via the Circle class. For example: Output: When you access an attribute via an …
Python Circle Class With Getter Setter Methods
Apr 24, 2021 · Write a Python program to design a Circle class with radius. Define a constructor, a setter setRadius() and a getter getRadius(). Write down a method to calculate area and the …
Python: A class constructed by a radius and two methods which …
Apr 21, 2025 · Write a Python class named Circle constructed from a radius and two methods that will compute the area and the perimeter of a circle. Sample Solution: Python Code: class …
- Some results have been removed