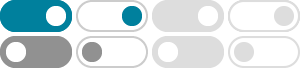
Getting Started With Testing in Python
Python has made testing accessible by building in the commands and libraries you need to validate that your applications work as designed. Getting started with testing in Python needn’t …
Effective Python Testing With pytest
pytest simplifies the testing workflow by allowing the use of normal functions and Python’s assert keyword, rather than relying on classes and specific assertion methods required by unittest. It …
Python's unittest: Writing Unit Tests for Your Code
In this tutorial, you'll learn how to use the unittest framework to create unit tests for your Python code. Along the way, you'll also learn how to create test cases, fixtures, test suites, and more.
Python's assert: Debug and Test Your Code Like a Pro
Jan 12, 2025 · In this tutorial, you'll learn how to use Python's assert statement to document, debug, and test code in development. You'll learn how assertions might be disabled in …
Python Testing Tutorials
Jan 18, 2025 · Learn how to test different types of Python applications, from command-line apps to web applications. Discover best practices and techniques for testing your Python …
How to Test Lambda Functions (Video) - Real Python
Python lambdas can be tested similarly to regular functions. It’s possible to use both unittest and doctest. The unittest module handles Python lambda functions similarly to regular functions. In …
Understanding the Python Mock Object Library
Jan 18, 2025 · To write a mock test in Python, you use the unittest.mock library to create mock objects and substitute them for real objects in your code, allowing you to test how your code …
4 Techniques for Testing Python Command-Line (CLI) Apps
In this article, you'll learn 4 essential testing techniques for Python command-line applications: "lo-fi" print debugging, using a visual debugger, unit testing with pytest and mocks, and integration …
Python's doctest: Document and Test Your Code at Once
In this tutorial, you'll learn how to add usage examples to your code's documentation and docstrings and how to use these examples to test your code. To run your usage examples as …
Python Type Checking (Guide) – Real Python
In this quiz, you'll test your understanding of Python type checking. You'll revisit concepts such as type annotations, type hints, adding static types to code, running a static type checker, and …