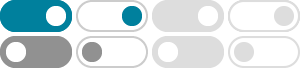
What's the fastest way to square a number in JavaScript?
What's the fastest way to square a number in JavaScript? function squareIt(number) { return Math.pow(number,2); } function squareIt(number) { return number * number; } Or some other …
What's the best way in JavaScript to test if a given parameter is a ...
Isn't this (Math.sqrt(number) % 1 === 0) basically enough? it just checks if the sqrt of the number is a whole number, if so, then it's a perfect square. Obviously, depending on what you want to …
Square each number in an array in javascript - Stack Overflow
May 26, 2014 · let array = [1,2,3,4,5]; function square(a){ // function to find square return a*a; } arrSquare = array.map(square); //array is the array of numbers and arrSquare will be an array …
javascript - Square every digit of a number - Stack Overflow
May 10, 2018 · //function takes number as an argument function convertNumber(num){ //the toString method converts a number into a string var number = num.toString(); //the split …
javascript - Find Nearest square number of a given number - Stack …
Apr 17, 2018 · The task is to find the nearest square number, nearest_sq(n), of a positive integer n (taken from codewars). I've tried the below but just get an infinite loop.
Javascript print square using for loop and conditional statement only
Oct 13, 2017 · % remainder operator, which returns a rest of a number which division returns an integer number. && logical AND operator, which check both sides and returns the last value if …
javascript - How do I square a number's digits? - Stack Overflow
Jun 16, 2015 · Write a function that returns the square of a number without using *,+ or pow Hot Network Questions Competing risks, Spline Coxph model, Plot PREDICTED Hazard Ratios on …
How to find square of n with a for loop javascript
Aug 24, 2021 · That corresponds to the first square. Next iteration corresponds to the first row, second column - etc, until i <= n is no longer fulfilled, at the end of the row. The next row then …
Javascript: Testing for perfect squares without using the inbuilt ...
Aug 6, 2018 · I am new to javascript and taking some tests on codewars, I am writing a code that checks for perfect numbers i.e. if a number has a squareroot that is a whole number it should …
How to square the value and sum together in javascript
Aug 21, 2018 · In Javascript, I have a scenario to implement the following: Let's take a list of integer below, take each integer in that list and double the value, then square the doubled …