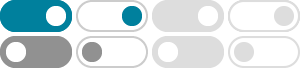
NumPy: the absolute basics for beginners — NumPy v2.2 Manual
As with built-in Python sequences, NumPy arrays are “0-indexed”: the first element of the array is accessed using index 0, not 1. Like the original list, the array is mutable. >>> a [ 0 ] = 10 >>> a array([10, 2, 3, 4, 5, 6])
numpy.array — NumPy v2.2 Manual
numpy.array# numpy. array (object, dtype = None, *, copy = True, order = 'K', subok = False, ndmin = 0, like = None) # Create an array. Parameters: object array_like. An array, any object exposing the array interface, an object whose __array__ method returns an array, or any (nested) sequence. If object is a scalar, a 0-dimensional array ...
NumPy quickstart — NumPy v2.2 Manual
There are several ways to create arrays. For example, you can create an array from a regular Python list or tuple using the array function. The type of the resulting array is deduced from the type of the elements in the sequences.
Array creation — NumPy v2.2 Manual
There are 6 general mechanisms for creating arrays: Conversion from other Python structures (i.e. lists and tuples) Intrinsic NumPy array creation functions (e.g. arange, ones, zeros, etc.) Replicating, joining, or mutating existing arrays. Reading arrays …
The N-dimensional array (ndarray) — NumPy v2.2 Manual
Arrays can be indexed using an extended Python slicing syntax, array[selection]. Similar syntax is also used for accessing fields in a structured data type.
numpy.where — NumPy v2.2 Manual
>>> import numpy as np >>> a = np. arange (10) >>> a array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]) >>> np. where (a < 5, a, 10 * a) array([ 0, 1, 2, 3, 4, 50, 60, 70, 80, 90]) This can be used on multidimensional arrays too:
Indexing on ndarrays — NumPy v2.2 Manual
ndarrays can be indexed using the standard Python x[obj] syntax, where x is the array and obj the selection. There are different kinds of indexing available depending on obj: basic indexing, advanced indexing and field access. Most of the following examples show the use of indexing when referencing data in an array.
numpy.zeros — NumPy v2.2 Manual
numpy.zeros# numpy. zeros (shape, dtype = float, order = 'C', *, like = None) # Return a new array of given shape and type, filled with zeros. Parameters: shape int or tuple of ints. Shape of the new array, e.g., (2, 3) or 2. dtype data-type, optional. The desired data-type for the array, e.g., numpy.int8. Default is numpy.float64.
Mathematical functions — NumPy v2.2 Manual
First array elements raised to powers from second array, element-wise. fmod (x1, x2, /[, out, where, casting, ...]) Returns the element-wise remainder of division.
numpy.dot — NumPy v2.2 Manual
numpy.dot# numpy. dot (a, b, out = None) # Dot product of two arrays. Specifically, If both a and b are 1-D arrays, it is inner product of vectors (without complex conjugation). If both a and b are 2-D arrays, it is matrix multiplication, but using matmul or a @ b is preferred.