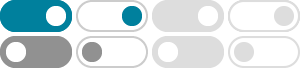
How do I represent and work with n-bit vectors in Python?
Apr 17, 2017 · class bit_vector(Structure): _fields_ = [('bits', c_uint32, 24), ('unused', c_uint32, 8), ] bv = bit_vector() # turn on the 18th bit -- being explicit just to demo it bv.bits |= …
A Bit Vector Class in Python - Purdue University
There are three ways to construct a bit vector with the class shown here: bv = BitVector( N ) which creates a bit vector that can hold N bits; or bv = BitVector( [1,0,1,0,0,1,0,1,0,0,1,0,1,0,0,1] ) …
What Is a Bit Vector? - Tech With Tech
Feb 28, 2022 · You’ll learn what bit vectors are, how they look, and what values their elements can take. Moreover, how to create them, display them, and do other operations with bit vectors …
BitArrays - Python Wiki
Bit arrays, bitstrings, bit vectors, bit fields. Whatever they are called, these useful objects are often the most compact way to store data. If you can depict your data as boolean values, and can …
BitVector - PyPI
May 30, 2021 · With regard to the basic purpose of the module, it defines the BitVector class as a memory-efficient packed representation for bit arrays. The class comes with a large number of …
Python: module BitVector - Purdue University
# Construct a bit vector from a list or tuple of bits: bv = BitVector.BitVector( bitlist = (1, 0, 0, 1) ) print bv # 1001 # Construct a bit vector from an integer: bv = BitVector.BitVector( intVal = 5678 …
C++ Writing Binary Vector and Reading it in Python
Jul 13, 2023 · What is a Binary Vector? A binary vector, also known as a bit vector or bit array, is a data structure used to store a sequence of binary values, typically represented as 0s and 1s. …
how to implement a really efficient bitvector sorting in python
Jun 7, 2010 · Using psyco speeds it up a little bit for me, but you can really speed it up by using a bit vector module written in C. I used bitarray and then the bit sorting method surpasses the …
How to represent a binary vector in Python efficiently
May 7, 2025 · I'm doing data analysis (e.g. using Local Binary Pattern) on Python and I'm trying to optimize my code. In my code I'm using binary vectors which are implemented currently as …
A Bit Vector Class in Python, Version 1.1 - Purdue University
There are five different ways in which you can construct a bit vector. (1): You can construct a bit vector directly from either a tuple or a list of bits, as in bv = BitVector( bits = …